Personal Finance Management Python: Build a Budget Tool
Personal finance management python is quite challenging. Many people can’t cope with the tracking of their income and expenses. But using all the proper tools, it’s really not a tough thing to get on top of your game of finances. In this guide, we will learn how to build a budget tool by using Python. This step-by-step guide will assist you with making your individual accounting the executives instrument, so your funds are on top.
Introduction to Personal Finance Management
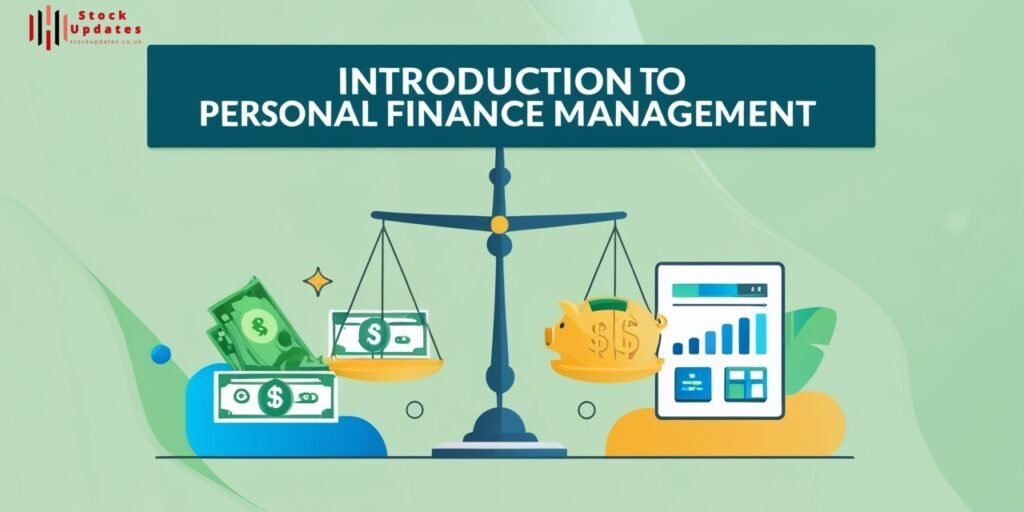
Personal financial management is the strategy for picking and controlling your monetary exercises. It embraces budgeting, saving, investing, and reasonable spending. Proper personal finance management guides your proper decision-making regarding your money.
Budgeting forms one of the main steps towards it. This refers to the distribution of income acquired towards different expenses, savings, and investments. From the budget, you determine your spending habits and what need to improve in certain areas.
There are many reasons to use Python as a personal finance management tool. First, Python is a powerful language that is at the same time user-friendly and versatile. It offers so many libraries designed for data analysis which make it suitable for financial data management.
Reason to Choose Python as a Personal Finance Management Tool?
There are several reasons why you should choose Python as your tool for personal finance management:
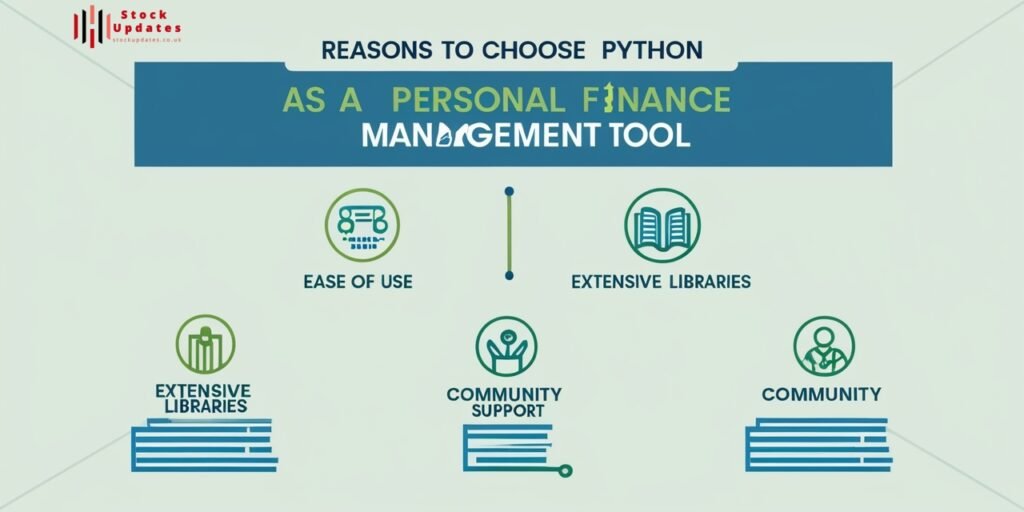
Ease of Use for Beginners
Python is one of the languages that are simple in syntax. This will enable people with little or no background in programming to be able to write in Python with just about no difficulty. Even if you do not have experience in programming, you can learn very easily the basics and start your budget tool.
Robust Libraries for Data Handling
Python has a huge area of libraries that support all types of data manipulation. Libraries such as Pandas and Matplotlib are perfect in handling financial data. Using Pandas, you can easily create and manipulate data structures, while Matplotlib allows you to put everything into perspective through visualization.
Community Support and Resources
The people group for Python is huge and exceptionally dynamic. If something is stopping you from building your tool, there are usually many resources online that you may use. You’ll find forums, tutorials, and documentation to solve the problem or learn some new technique.
Setting Up Your Python Environment for Personal Finance Management
To get everything rolling, you should set up your Python climate in the event that you haven’t as of now. You need to do this.
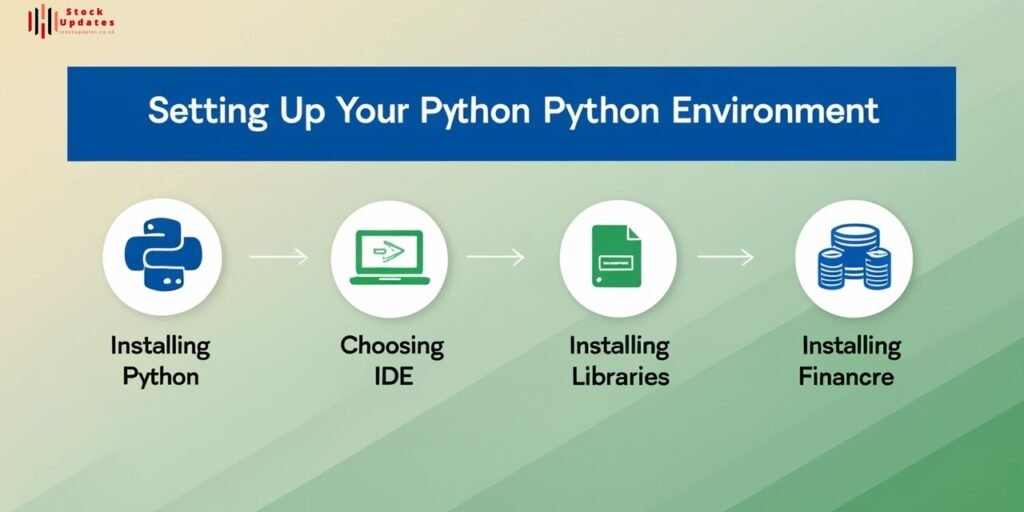
Installation of Python
First, you must install Python from your computer. Download the latest available version of Python by visiting Python Official Site and then proceed with the installation according to your chosen operating system.
Recommended IDEs for Coding
An Integrated Development Environment is what will help you code much more easily. Here are some recommended IDEs for the language:
- PyCharm: It is a pretty powerful IDE for Python development with quite many features.
- Visual Studio Code: It is a lightweight code supervisor, lightweight yet flexible.
- Jupyter Notebook: The IDE is specifically used for data analysis and visualization.
Select a suitable IDE according to your requirement and then download it.
Installing Required Libraries
You will need some libraries to create your budget tool. Open your order brief/terminal and introduce the accompanying libraries utilizing pip:
bash
pip install pandas matplotlib
Creating Data Model for Budget Tool in Personal Finance Management Python
One of the central features of your budget tool will be the ability to store and manipulate data. Toward that end, we’ll employ a Pandas DataFrame to represent your financial data. Let’s start by establishing a simple structure.
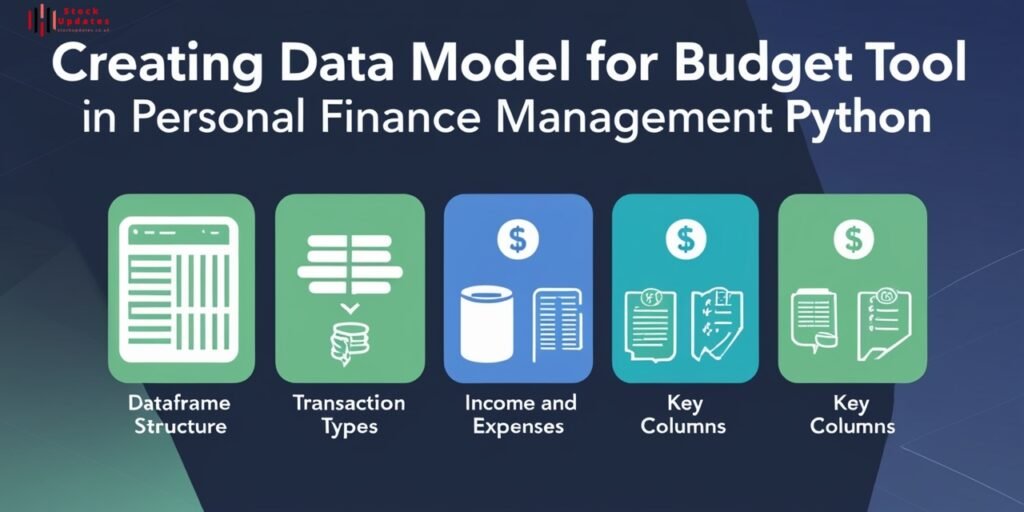
Understanding Data Structures in Personal Finance Management Python
In Python, a DataFrame is a two-layered, size-impermanent, and possibly heterogeneous even information structure. It can be considered as a spread sheet or SQL table. You can contemplate a DataFrame by organizing your monetary information into lines and sections.
Using Pandas to Create a DataFrame
Here’s how to go about initializing an empty DataFrame for the budget tool.
Python
import pandas as pd
# Initialize an empty DataFrame
columns = ['Date', 'Type', 'Category', 'Amount']
finance_data = pd.DataFrame(columns=columns)
This DataFrame holds four types of primary data criteria for every transaction: date, type (income/expense), category, and amount.
Defining Key Columns
Every segment in the DataFrame is utilized for a particular reason:
- Date: Date of the transaction
- Type: Whether it is an income or an expense
- Category: Food, transportation, entertainment, etc.
- Amount: The monetary or number value of the transaction.
Building User Interface of Personal Finance Management Python
The following thing you need to accomplish for your financial plan device is making a user interface (UI). For this venture, we’ll fabricate a straightforward order line interface. It will assist users with effectively entering their pay and costs.
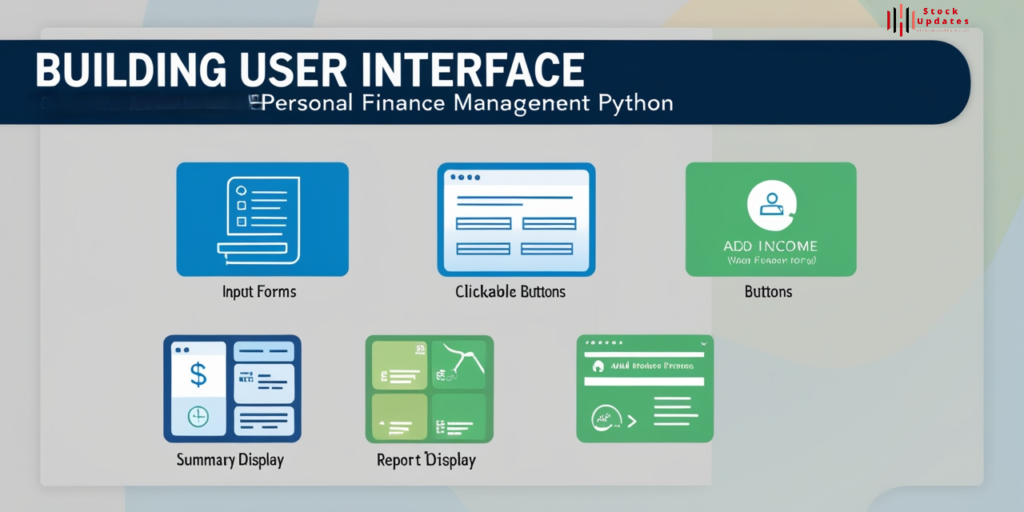
Types of Options of User Interface
You may choose different kinds of user interfaces:
- Command-Line Interface (CLI): The user interface is AI text based interface and uses commands.
- Graphic User Interface (GUI): It’s a graphical interface with buttons and forms.
For simplicity, let’s start with CLI.
Building a Command-Line Interface
You can build a simple menu for your budget tool like this:
Python
def display_menu():
print("Personal Finance Tool")
print("1. Add Income")
print("2. Add Expense")
print("3. Generate Report")
print("4. Exit")
Accepting User Input for Income and Expenses
Finally, design functions for handling user’s input. Using these functions, users can enter income or expenditure:
Python
def add_income(data):
date = input("Enter date (YYYY-MM-DD): ")
category = input("Enter income category: ")
amount = float(input("Enter amount: "))
new_row = {'Date': date, 'Type': 'Income', 'Category': category, 'Amount': amount}
return data.append(new_row, ignore_index=True)
def add_expense(data):
date = input("Enter date (YYYY-MM-DD): ")
category = input("Enter expense category: ")
amount = float(input("Enter amount: "))
new_row = {'Date': date, 'Type': 'Expense', 'Category': category, 'Amount': -amount}
return data.append(new_row, ignore_index=True)
Implementing Core Features of Your Budget Tool
Since we have our essential arrangement, how about we include some center elements. These will enable users to add transactions and even view their budget.
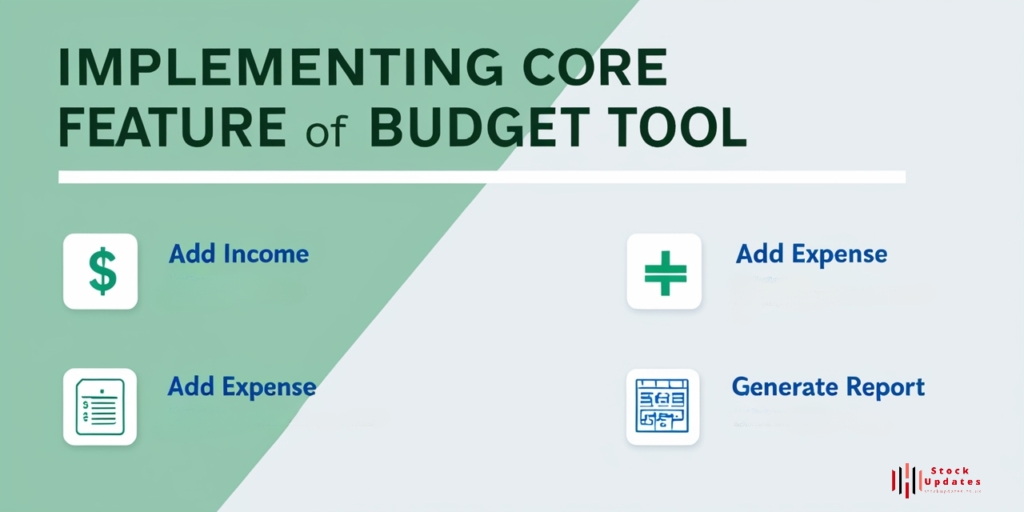
Adding Transactions
A user will be able to add transactions by the selection of options from the menu. For example:
Python
def main():
finance_data = pd.DataFrame(columns=['Date', 'Type', 'Category', 'Amount'])
while True:
display_menu()
choice = input("Choose an option: ")
if choice == '1':
finance_data = add_income(finance_data)
elif choice == '2':
finance_data = add_expense(finance_data)
elif choice == '3':
generate_report(finance_data)
elif choice == '4':
break
else:
print("Invalid choice. Please try again.")
Viewing Current Budget
To Check the current budget, we have to create the summary report function. This function prints out both the income and expenses:
Python
def generate_report(data):
income = data[data['Type'] == 'Income']['Amount'].sum()
expenses = data[data['Type'] == 'Expense']['Amount'].sum()
balance = income + expenses
print("\nSummary Report:")
print(f"Total Income: {income}")
print(f"Total Expenses: {expenses}")
print(f"Balance: {balance}")
Advanced Features in Personal Finance Management Python
Now that you have a minimal budgeting tool, take some time to think about implementing some advanced features. These can enhance the functionality and usability of your tool.
Data Visualization using Matplotlib
Graphical representation of your financial data may help you understand where you spend most of your money. Use Matplotlib to create charts that represent your income and expenses.
Python
import matplotlib.pyplot as plt
def plot_data(data):
categories = data['Category'].value_counts()
categories.plot(kind='bar')
plt.title('Expenses by Category')
plt.xlabel('Category')
plt.ylabel('Amount')
plt.show()
Configuring Automated Budget Alerts
You can automatically track your budget by automating alerts. For example, you can set a threshold for each category and be notified when the threshold is reached.
Using Machine Learning to Analyze Expenses for Projections
Advanced users can even apply machine learning to identify any typical spending habits. With Scikit-learn libraries, you can do future expense prediction given historical data.
Testing and Debugging Your Budget Tool in Personal Finance Management Python
Testing is absolutely essential for testing that your tool works right. Here is the list of tips to test your budget tool:
Common Issues and Solutions
While developing your tool, some of the common issues might pop up:
- Incorrect Data Types: Verify that you are using the right data types, especially with quantities.
- Index Errors: Be careful of indexing while appending or accessing DataFrame rows.
Best Practices for Testing Python Code
- Test Cases: Each function should be written under test to determine if it works.
- Debugging: Take printed statements or use debugger tools to find the bugs.
Conclusion: Mastering Personal Finance Management Python
This article directed you through how to construct a planning device utilizing Python so you can begin assuming command over your individual budgets. You will have powerful insights into your spending habit and the potential for change in your life by keeping effective control of your income and expenses. The flexibility of Python allows you to make the tool you will use fit exactly your needs in handling personal finance.
As you build further on your budgeting tool, consider data visualization or even automated alerts. Such information can be stored for easier management of your finances, thus keeping you on the right track toward achieving your goals. Build your budget tool today and have fun taking advantage of organized finances and better decision-making!
Must read Random Stock Generator Python Code.
Read more about Personal Finance at Stock Updates.
1 comment